
NestJS介绍
Nest.js是一个渐进的Node.js框架。它的核心思想是提供了一个层与层直接的耦合度极小、抽象化极高的一个架构体系。
-
为什么使用NestJS
起初没打算使用Node来做,但得知了NestJS之后,忍不住想要试一试,于是就使用了NestJS。 -
NestJS中文网 https://www.itying.com/nestjs/
-
在学习NestJS之前还需要了解TS相关 https://www.tslang.cn/docs/home.html
-
NestJS使用体验
NestJS就如介绍所说,耦合度低,层次分明,使用起来还挺好上手的。 -
安装(NodeJS环境)
如果你不知道怎么安装Node可以看中文网 http://nodejs.cn/download/
下载对应的文件,然后安装即可。
1 | // 检查版本 |
使用 Nest CLI 建立新项目非常简单
1 | yarn global add @nestjs/cli |
创建后的主要文件简介
1 | app.controller.ts //带有单个路由的基本控制器示例 |
main.ts 入口文件,定义了请求端口,初始化的模块
1 | import { NestFactory } from '@nestjs/core'; |
主要文件作用及含义
- article.controller.ts
提供接口,供客户端调用,这里仅提供接口,逻辑层在app.service.ts编写
- article.service.ts
业务逻辑层,主要与数据库交互,操作数据库
- article.module.ts
引入数据库表,注入依赖项(server),控制器等
- article.interface.ts
定义接口数据
- dto/create-post.dto.ts
将定义数据会怎样发送到网络,名称首字母需要大写,否则存入不到数据库
- schemas/article.schema.ts
定义将存储在数据库中的数据类型 与客户端信息json一致
nest创建指令
1 | // 模块 |
使用mongodb数据库
安装
1 | cnpm install --save @nestjs/mongoose mongoose |
- 在app.module.ts中连接数据库
1 | // 这里记得mongodb服务是打开的 |
article.module.ts使用
1 | import { MongooseModule } from '@nestjs/mongoose'; |
配置article.schema
1 | // 定义将存储在数据库中的数据类型 与客户端信息json一致 |
以上就是连接数据库的过程,这里需要注意mongodb的安装及启用,这里不再具体说明,可以自行百度。
服务层service.ts
1 | import { Injectable } from '@nestjs/common'; |
控制器定义api接口
1 | import { Controller, Post, UseGuards, Body, Get, HttpStatus, Res, Query } from '@nestjs/common'; |
关于问题
由于这个博客已经做了快一个月了吧,当时也没想着写总结,所以有些问题其实已经记不大清了,如果有什么问题可以发送邮箱,共同讨论。
以上是搭建博客中台的基本过程,由于是第一次使用NestJS,踩的坑不多也不少,总之整个过程还算顺利,而且自己写前后端其实是很爽的,做个人项目的话NestJS也算是比较合适的服务端框架了,上手较快,学习成本还算可以,很适合用来练手。第一次使用还是有很多不足的,在这里感谢https://www.itying.com/nestjs/ 这个中文网,这个文档还是挺棒的。
本博客移动端(Android)下载:https://www.pgyer.com/XnUz
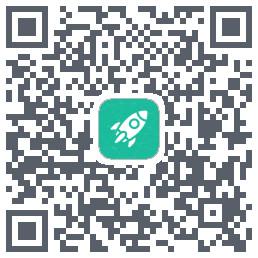
- 本文标题:Nest搭建中台服务
- 本文作者:sonder
- 创建时间:2020-06-30 14:33:32
- 本文链接:https://sonderss.github.io/2020/06/30/Nest搭建中台服务/